In this blog you will Learn how to Show post list with load more posts (ajax) functionality.
For this you will create a template file for post and set the template to page where you need to show posts, and also you will set ajax code for show more posts on button click without refresh or redirect to page.
There are many plugins also available that provide the same functionality you can do but in this blog you will learn how this can be done by using your code.
Step1: Create Template page.
Let’s first create Template page inside your theme or child theme. you can create template by adding file to root folder of your theme. create file with name showposts.php and add code like below.
<?php /* Template Name: Show Posts Template Post Type: post, page, event */ // Page code here...
This will set template page and now you can select it to page in WordPress admin create page view into template section. and at place of page code we will set page.php code so it will get header and footer same as theme.
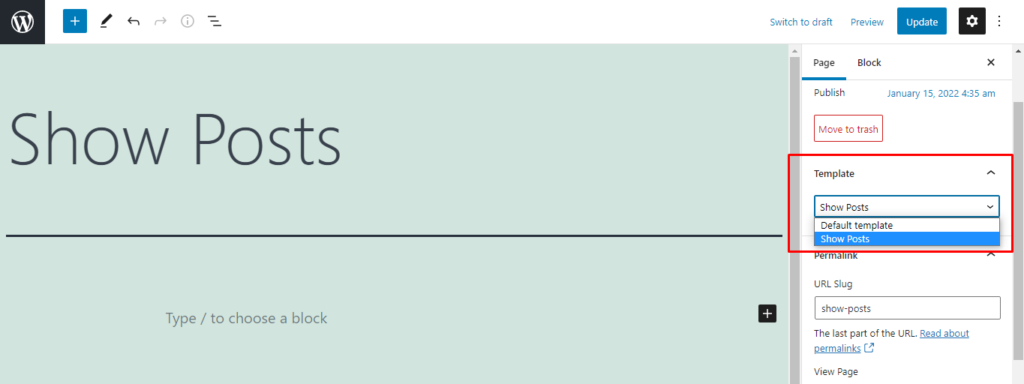
Now this will show template page content to page, lets add an ajax call for getting post.
Step2: Create Container div for hold post inside.
Let’s create a block for holding posts inside it and also a button for loading more post-event. you need to give a data-page attribute for set page number and it will increase based on response and posts count.
<?php /* Template Name: Show Posts ... */ ... <div id="postslist-container" data-page="1"></div> <div class="buttonContainer"><button type="button" class="loadmorepost">Load More</button></div> ...
Step3: Ajax call to admin ajax function.
Let s create ajax call in javascript. you need to add this on same template page you are working on.
... ?> <script type="text/javascript"> ... var page = jQuery('#postslist-container').attr('data-page'); page = page ? page : 1; jQuery.ajax({ type: "post", dataType: "json", url: "<?php echo admin_url('admin-ajax.php'); ?>", data: {action: 'themeprefix_showmoreposts', page: page}, success: function(response){ console.log(response); } }); ... </script> <?php ...
Step4: Define Callback function for ajax call.
To define the callback function you need to write it on the function.php file of the active theme. here in this callback, the function you will need to write a query for getting posts based on page passed from the ajax post-call.
you can write it inside theme or plugins file or you should have child theme of your active theme and write your extra code there so it will prevent for data lost. please refer one of our blog for how to create child theme
add_action( 'wp_ajax_themeprefix_showmoreposts', 'themeprefix_showmoreposts_content_callback' ); add_action( 'wp_ajax_nopriv_themeprefix_showmoreposts', 'themeprefix_showmoreposts_content_callback' ); function themeprefix_showmoreposts_content_callback(){ $returnArray = array('status' => false); $posts_per_page = 10; global $wpdb; if($_POST['page'] != ''){ $paged = $_POST['page']; $haveMorePost = false; $htmlstring = ''; $get_posts_query = new WP_Query( array( 'post_type' => 'post', 'post_status' => 'publish', 'posts_per_page' => $posts_per_page, 'paged' => $paged, ) ); ob_start(); if($get_posts_query->found_posts >= $posts_per_page){ $haveMorePost = true; } while ( $get_posts_query->have_posts() ) : $get_posts_query->the_post(); echo '<div class="postItem" style="margin-bottom:10px;font-size:13px;">'; echo '<h4 style="margin-bottom:10px">'.get_the_title().'</h4>';//get_template_part('template-parts/content', 'post', true); echo '<div class="postContent">'.get_the_excerpt().'</div>'; echo '<a href="'.get_the_permalink().'" >View</a>'; echo '</div>'; endwhile; // get output to variable $htmlstring = ob_get_clean(); $returnArray = array( 'status' => true, 'html' => $htmlstring, 'haveMorePost' => $haveMorePost ); }else{ $returnArray = array( 'status' => false, 'message' => 'page paramter not found', ); } // return json response to ajax call wp_send_json($returnArray); exit; }
Now you need to handle returned JSON responses in the ajax call response function.
<script type="text/javascript"> function getPosts(){ var page = jQuery('#postslist-container').attr('data-page'); page = page ? page : 1; // start loading jQuery('#postslist-container').addClass('loading'); jQuery('.loadmorepost').text('Loading...'); // ajax call jQuery.ajax({ type: "post", dataType: "json", url: "<?php echo admin_url('admin-ajax.php'); ?>", data: {action: 'themeprefix_showmoreposts', page: page}, success: function(response){ console.log(response); // if get post add html to container if(response.status == true){ jQuery('#postslist-container').append(response.html); if(response.haveMorePost == true){ jQuery('#postslist-container').attr('data-page', parseInt(page) + 1); }else{ jQuery('.loadmorepost').hide(); } } // stop loading jQuery('#postslist-container').removeClass('loading'); jQuery('.loadmorepost').text('Load More'); } }); } jQuery(document).ready(function(){ getPosts(); }); jQuery(document).on('click','.loadmorepost', function(){ getPosts(); }) </script>
here, we have put our ajax call into a function and called it 2 time one when the page is loads and second when user clicks on load more button. so both time our function will get page number from data-page attribute of postslist-container div and call ajax callback function for get that page posts.
after get response from ajax callback function it will append returns html to postlist-container div.
here you can find full code for template page file and ajax callback function in function.php file. https://github.com/gvtechnolab-in/ajax-load-more-posts.git
Thankyou for reading now you can Show post list with load more posts (ajax) functionality and also you can improve by using it for different post or filter attributes also.